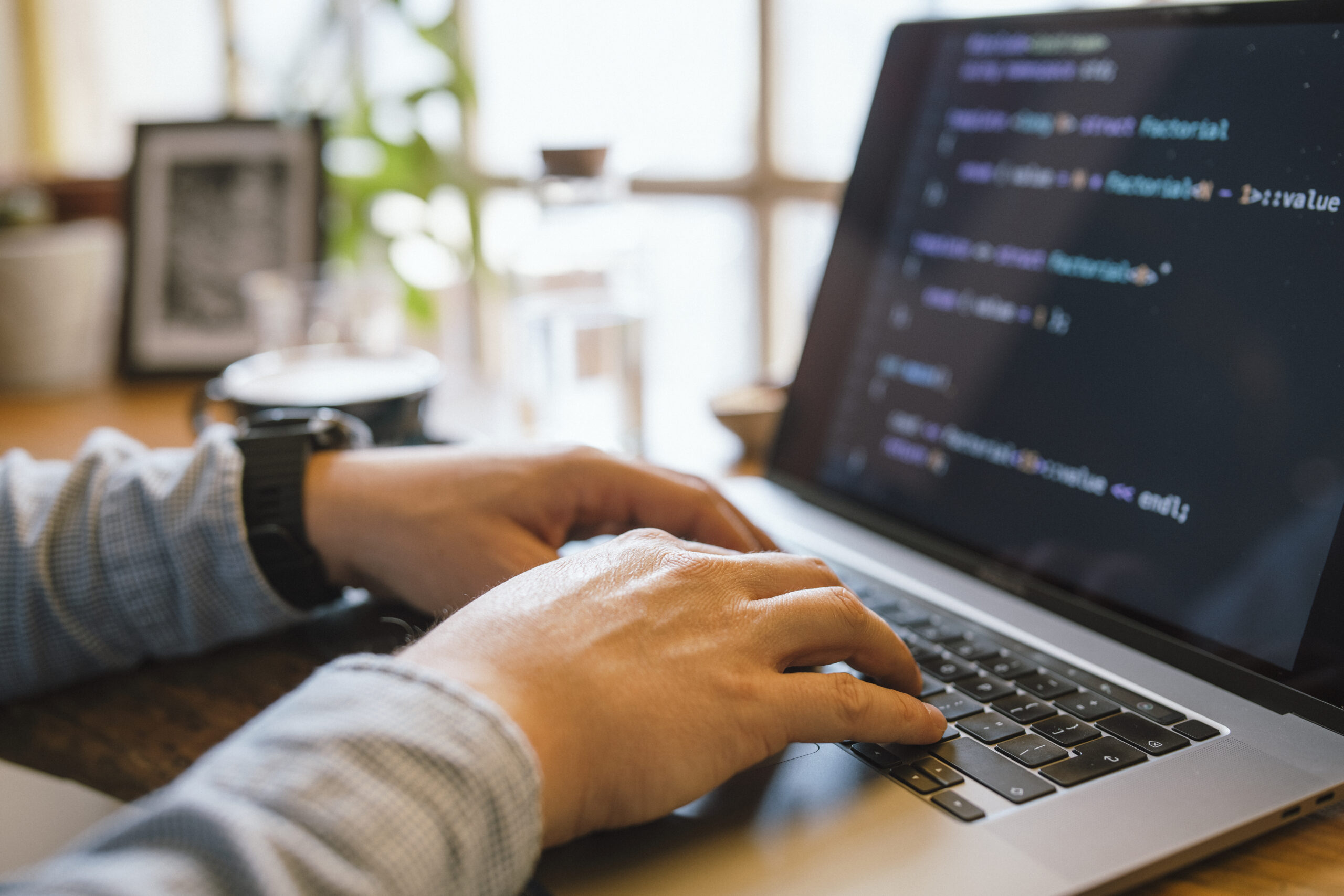
Debugging is one of the most crucial — still often ignored — expertise in a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Erroneous, and Discovering to Imagine methodically to unravel problems efficiently. Whether or not you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save several hours of annoyance and substantially boost your productiveness. Listed below are numerous methods to assist developers degree up their debugging sport by me, Gustavo Woltmann.
Learn Your Applications
On the list of fastest means builders can elevate their debugging capabilities is by mastering the resources they use each day. While crafting code is one Element of progress, being aware of the best way to interact with it proficiently for the duration of execution is Similarly crucial. Modern progress environments arrive equipped with impressive debugging abilities — but a lot of developers only scratch the area of what these resources can perform.
Just take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code on the fly. When applied properly, they let you notice exactly how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer equipment, including Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on network requests, look at serious-time functionality metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can transform aggravating UI problems into manageable responsibilities.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging processes and memory management. Mastering these tools could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model control techniques like Git to be aware of code record, find the exact moment bugs ended up released, and isolate problematic variations.
Ultimately, mastering your tools implies heading over and above default configurations and shortcuts — it’s about acquiring an personal expertise in your development atmosphere to make sure that when issues come up, you’re not dropped at nighttime. The higher you recognize your equipment, the more time you'll be able to expend resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
One of the more significant — and infrequently neglected — methods in successful debugging is reproducing the trouble. Ahead of jumping into the code or making guesses, builders need to have to make a steady setting or situation exactly where the bug reliably seems. With no reproducibility, repairing a bug gets to be a activity of chance, generally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as you can. Ask thoughts like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or output? Are there any logs, screenshots, or mistake messages? The greater depth you have, the easier it will become to isolate the exact circumstances less than which the bug happens.
Once you’ve collected enough data, attempt to recreate the issue in your neighborhood atmosphere. This might imply inputting the exact same information, simulating very similar consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automated exams that replicate the sting situations or point out transitions concerned. These assessments not only support expose the problem but in addition avoid regressions Down the road.
Occasionally, The problem can be environment-certain — it'd take place only on specified functioning systems, browsers, or below unique configurations. Using resources like virtual equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a state of mind. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continuously recreate the bug, you're currently halfway to repairing it. That has a reproducible state of affairs, You may use your debugging applications more effectively, test possible fixes safely and securely, and converse far more Plainly using your staff or end users. It turns an abstract grievance right into a concrete problem — and that’s exactly where developers prosper.
Browse and Have an understanding of the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes wrong. Rather than looking at them as discouraging interruptions, developers should really study to deal with error messages as immediate communications with the process. They typically show you just what exactly took place, exactly where it happened, and in some cases even why it took place — if you know how to interpret them.
Start by studying the information thoroughly and in full. Quite a few developers, specially when underneath time force, glance at the main line and quickly begin creating assumptions. But further inside the mistake stack or logs may perhaps lie the real root trigger. Don’t just duplicate and paste error messages into search engines like google and yahoo — read through and comprehend them to start with.
Crack the error down into sections. Is it a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it position to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to grasp the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Mastering to acknowledge these can greatly quicken your debugging course of action.
Some faults are vague or generic, As well as in Those people instances, it’s critical to examine the context through which the mistake occurred. Verify relevant log entries, enter values, and recent adjustments from the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger concerns and provide hints about probable bugs.
Finally, error messages are certainly not your enemies—they’re your guides. Discovering to interpret them properly turns chaos into clarity, aiding you pinpoint troubles more rapidly, lower debugging time, and turn into a extra efficient and self-confident developer.
Use Logging Sensibly
Logging is One of the more powerful tools inside a developer’s debugging toolkit. When employed properly, it provides serious-time insights into how an software behaves, encouraging you understand what’s happening under the hood without having to pause execution or step in the code line by line.
A good logging method begins with understanding what to log and at what level. Typical logging ranges include DEBUG, INFO, Alert, Mistake, and Lethal. Use DEBUG for specific diagnostic facts through progress, Details for standard functions (like productive commence-ups), WARN for possible issues that don’t crack the appliance, ERROR for actual complications, and Deadly once the program can’t carry on.
Avoid flooding your logs with too much or irrelevant knowledge. A lot of logging can obscure essential messages and decelerate your program. Concentrate on crucial events, point out adjustments, enter/output values, and significant choice details with your code.
Format your log messages Plainly and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even much easier to parse and filter logs programmatically.
Throughout debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in production environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. Using a very well-thought-out logging technique, you can reduce the time it will require to identify problems, achieve further visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a sort of investigation. To correctly determine and resolve bugs, builders ought to approach the process like a detective fixing a secret. This mindset assists break down intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Get started by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, obtain just as much suitable information and facts as you can without having jumping to conclusions. Use logs, examination scenarios, and person experiences to piece collectively a clear image of what’s taking place.
Subsequent, type hypotheses. Ask your self: What might be creating this behavior? Have any improvements not long ago been manufactured on the codebase? Has this concern transpired prior to below comparable circumstances? The goal should be to narrow down alternatives and detect probable culprits.
Then, examination your theories systematically. Make an effort to recreate the issue in a managed surroundings. In the event you suspect a specific functionality or part, isolate it and verify if the issue persists. Just like a detective conducting interviews, inquire your code queries and let the effects direct you nearer to the truth.
Fork out near attention to smaller particulars. Bugs normally conceal in the minimum expected destinations—just like a lacking semicolon, an off-by-one particular error, or possibly a race situation. Be extensive and affected person, resisting the urge to patch The difficulty with out thoroughly comprehending it. Temporary fixes may well hide the true trouble, only for it to resurface later on.
Lastly, hold notes on Anything you attempted and figured out. Just as detectives log their investigations, documenting your debugging process can preserve time for upcoming problems and enable others realize your reasoning.
By imagining like a detective, developers can sharpen their analytical capabilities, method troubles methodically, and come to be more effective at uncovering hidden troubles in elaborate systems.
Compose Assessments
Producing checks is one of the best solutions to improve your debugging abilities and All round progress performance. Checks not only help catch bugs early but additionally serve as a security Web that offers you assurance when making modifications for your codebase. A effectively-examined application is easier to debug since it permits you to pinpoint accurately where and when a problem occurs.
Get started with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can speedily expose website whether a selected bit of logic is Performing as predicted. Each time a check fails, you instantly know exactly where to look, significantly lessening the time used debugging. Device exams are Particularly helpful for catching regression bugs—issues that reappear just after Earlier getting set.
Future, combine integration exams and stop-to-finish checks into your workflow. These enable be certain that numerous aspects of your software function together efficiently. They’re specifically useful for catching bugs that occur in complex devices with several factors or expert services interacting. If one thing breaks, your checks can let you know which part of the pipeline unsuccessful and under what ailments.
Creating tests also forces you to definitely Believe critically regarding your code. To test a element effectively, you would like to grasp its inputs, envisioned outputs, and edge circumstances. This volume of comprehension naturally qualified prospects to better code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. When the test fails persistently, you could give attention to correcting the bug and view your take a look at go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
To put it briefly, creating exams turns debugging from the disheartening guessing match right into a structured and predictable process—aiding you catch additional bugs, a lot quicker and much more reliably.
Get Breaks
When debugging a difficult challenge, it’s quick to become immersed in the issue—looking at your monitor for several hours, trying Answer right after Remedy. But Among the most underrated debugging instruments is solely stepping absent. Having breaks allows you reset your intellect, cut down frustration, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive fatigue sets in. You might start overlooking obvious faults or misreading code that you choose to wrote just several hours earlier. In this point out, your Mind will become considerably less productive at difficulty-solving. A short wander, a espresso split, or perhaps switching to a different endeavor for ten–15 minutes can refresh your aim. Quite a few builders report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, In particular during extended debugging periods. Sitting before a display, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Vitality and a clearer way of thinking. You could suddenly detect a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb will be to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use that time to move all over, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In brief, getting breaks isn't an indication of weakness—it’s a wise system. It gives your brain House to breathe, improves your viewpoint, and can help you avoid the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and rest is an element of solving it.
Understand From Each Bug
Each and every bug you face is a lot more than simply a temporary setback—It really is a chance to mature as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or simply a deep architectural issue, each one can educate you anything valuable should you make time to mirror and review what went Completely wrong.
Start by asking your self several essential inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like unit testing, code critiques, or logging? The answers often reveal blind places in the workflow or understanding and help you build stronger coding patterns going ahead.
Documenting bugs can even be a fantastic routine. Retain a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see designs—recurring concerns or typical mistakes—that you can proactively stay clear of.
In staff environments, sharing Whatever you've discovered from the bug with the peers may be Specifically powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll begin appreciating them as critical areas of your development journey. In spite of everything, a few of the finest developers are certainly not the ones who publish perfect code, but individuals who continuously understand from their mistakes.
In the long run, each bug you resolve provides a whole new layer to your skill established. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It helps make you a far more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.